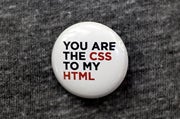
I've already discussed how you can learn to code for free using languages such as Ruby and JavaScript, but this time we'll explore an even more basic language that can help to make your websites pop. Cascading Style Sheets is like HTML's cooler, more artistic sibling: While HTML handles the structure and content of your website, CSS allows you to add cutting-edge design to it. This guide tells you everything you need to know to get started making better-looking websites fast.
What Is CSS?
Many people just getting to know CSS think of it as a fancy way of handling HTML properties such as text formatting and background images--and for small-scale examples on simple pages, that's largely true. You can manipulate many of the properties that CSS affects through pure HTML instead (though not as well), and if you're just looking to change the font size on your page, CSS is actually more difficult to use than HTML.What makes CSS worthwhile is the number of design options it opens up for you that are difficult--or impossible--to accomplish with HTML alone. HTML gives you just a handful of text properties to manipulate; CSS gives you properties such as
color
, direction
, letter-spacing
, line-height
, text-align
, text-decoration
, text-indent
, text-shadow
, text-transform
, vertical-align
, white-space
, and word-spacing
. And I haven't even gotten to the nontext options.
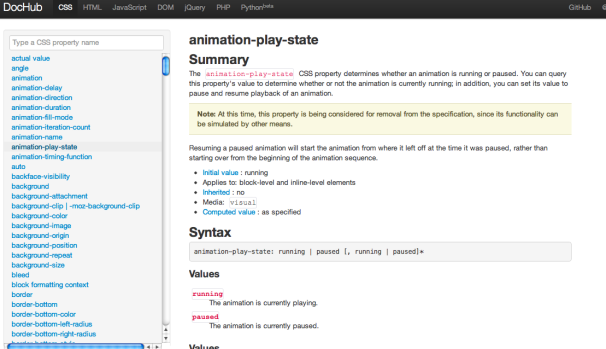
For a full list of CSS properties, you can check out a handy glossary on Dochub, but for now let's look at just two of the more interesting ones. Since we couldn't actually write HTML and CSS code in a Web page without causing trouble for the PCWorld CMS, we've taken the liberty of providing images of some example code and what it looks like on a live page.
For starters, CSS allows you to add and modify a border for any element on a page. The following code produces a solid, 5-pixel-wide red border around the whole paragraph.


CSS experts will note that there is a much shorter way to write this code (they'll also frown on writing the code inline at all), but it provides a good example of CSS formatting.
You write inline CSS in the tag itself, using the
style
attribute. Basically you write your HTML tags as usual, and then add CSS in the format "css-property: property-value;" right inside. Any arbitrary CSS can go inside the style
attribute. For instance, if we wanted to add a drop shadow to that
paragraph in addition to the border, we'd change the code to the
following line.

You may have noticed that the
box-shadow
attribute we added has more than one value. That's because the box-shadow
attribute allows for shorthand formatting, another timesaving feature
of CSS. In shorthand formatting, instead of specifying each related
value of a property individually, you can specify the values in a
standardized order to save time and characters. In the example above, we
specify the height of the drop shadow, its width, and its color.The
border
attribute has a similar shorthand, and we
could save a lot of space by writing our code in the following format,
which would produce the same results.

These cool visual tricks are really just the window dressing of CSS. The language's true strength comes from its ability to quickly and effectively target style effects to any part of your website thanks to powerful selectors. Learning CSS also allows you to standardize your design across multiple pages on your site using a single, easily modifiable external style sheet. Both of these features take months or years to fully master, but I can quickly demonstrate how and why you'd use CSS with a few simple examples.
External Styling and Selectors
Advanced users of CSS rarely add their styling to an individual page. Instead, veteran users deploy CSS through an external .css file that each page refers to as needed.I can't show you a direct example of this trick right now (for some reason, PCWorld's IT staff doesn't want a random editor mucking about with the code that runs the whole site), but I can show you the .css file we already use. Warning: Extremely boring link. That CSS file may not look like much, but every article generated on PCWorld refers to it to determine the default PCWorld page styles.
If we suddenly decided that we wanted every single PCWorld article to start showing its text as bright green (because we'd all lost our minds), applying that new hue to every article on the site would merely require us to change the text color of the
body
tag to green in that .css file.Of course, the problem with handling all your styling through one top-level document is that styling individual sections of a specific page can become tricky. For example, let's say you want to change how your headings look on one page but not on another. How would you accomplish that using CSS?
Well, CSS offers two options. The simpler but less flexible way is to add some CSS to that single page. By default, CSS code on an individual page overrides the CSS in an external .css file. So if your style.css says the default font size is 12px but the page itself indicates 24px text, the page in question will take that 24px as gospel.
That's great for making one individual change, but you have other ways to subvert your master .css file if you want to make changes to specific kinds of headlines that appear on, say, one in ten pages on your site. As your site grows, managing each individual page becomes more and more annoying. That's why we have classes and IDs. You can add a class and ID tag to almost any HTML element on a page to further specify its identity to your style sheet. Generally they look something like the following:


Your classes and IDs can't have spaces, but otherwise you can name them whatever you want. It may seem like a waste of time, but you want to bother adding these specifying tags because CSS can recognize them and use them to target only the tags that have them. If you want to make some of your headlines larger and red, for instance, you can give them all the class "BigRed" and then apply a style only to that class.
To understand how, you have to keep in mind that the formatting of CSS in a separate style sheet is slightly different than it is as an inline property. Since any given style you write isn't attached to a specific HTML tag, it needs a selector to identify the page elements to which you're attaching that style. CSS in a style sheet looks like this:
selector {attribute: value; attribute: value; attribute: value;}
So if we wanted to make all the text in the body of a Web page red, for instance, we would write
body {color: red;}
in our CSS stylesheet. That statement indicates that all the text inside of the body
tag on our Web pages should be colored red. The red
attribute works because red happens to be one of the colors you can
identify by name in CSS, but you can also use the hex value of a color (#ff0000
) or the RGB value (255,0,0
) to be more specific in your color choices.You can use the selector attribute to edit the styling of any HTML tag on your page, and then get even more granular by adding in a class or ID. When you're programming with CSS, you identify a class by using a period. If you want to select all elements with the class BigRed, for instance, you would write
.BigRed
as the selector. Meanwhile, you select an ID by using the pound symbol. For example, you would use #BigTitle
for an element with the ID BigTitle.In addition, you can chain together selectors to achieve even more specificity. If you wanted to select only paragraph tags with the class BigRed, excluding any headings or other HTML elements that have that class, you would write
p.BigRed
as your selector.Let's say we wanted to add our border to any paragraph that has the class BigRed. We'd throw the following line into our style sheet.
p.BigRed {border: solid 5px red;}
And now any paragraph with that class specified on any page linked to that style sheet would have the border added to it.
Since more-specific selectors take precedence over more-general ones, our selector using a class overwrites the styling for paragraphs in general. IDs are even more specific than classes (you can have only a single element on a page with any given ID). Say that we wrote the following line:
p#MostSpecific {border: solid 6px red;}
With this line in place, any paragraph with the ID MostSpecific would have a 6-pixel border rather than a 5-pixel border, even if it had the BigRed class.
Great Artists Steal
Of course, the easiest way to learn CSS is to start playing around with the code yourself. Although this simple explainer might be confusing without prior coding experience, it makes a lot of sense in context. For further help, I recommend that you check out a few of the great online resources for learning CSS. I'm a fan of the video tutorials on Don't Fear the Internet and the previously mentioned glossary of CSS tags on Dochub for general reference.
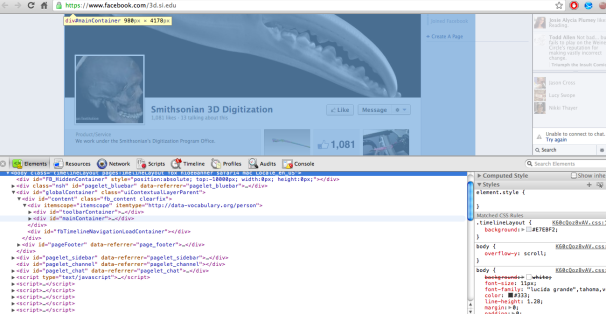
The best way to learn how to write great CSS, however, is to look at how other coders do it. Luckily, that's a simple exercise in practically any modern browser. Both Chrome and Safari come with built-in developer tools that let you quickly and easily look at the CSS, HTML, and JavaScript on any page. Firefox and Internet Explorer users have access to similar tools through the free extension Firebug. These tools make it easy to see the code that creates your favorite websites, even highlighting on the page whichever element you are currently mousing over in the page's source code, and vice versa.
The next time you see a layout or formatting trick that you really like on a website, you can feel confident firing up your browser's developer tools to take a look and maybe try it out for yourself. With a little curiosity and practice, you'll soon be able to understand and replicate tricks from even the most beautiful sites on the Web.
Ref:
Pcword
No comments:
Post a Comment